Getting Shit Done
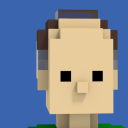
[Edit]: There's a better, live branch at GitHub. Use leftnode's version.
Here's an archive of my changes before I discovered leftnode's branch.
- The syntax errors were fixed. (Like the "play" string in the dictionary at the end that was supposed to be the play method.)
- It's more Windows friendly. Changing the "hosts" file doesn't require a network driver restart in Windows.
- You no longer have to specify an argument, "work" or "play." It'll just toggle modes every time you run it now.
- When reverting to "play" mode, it retains whatever was in the hosts file after the endToken now.
- I removed the bit of code that optionally reads in from an ini file. The ini file format (the keys, in particular) struck me as awkward.
#!/usr/bin/env python import sys import getpass import subprocess import os def exit_error(error): print >> sys.stderr, error exit( 1 ) restartNetworkingCommand = ["/etc/init.d/networking", "restart"] # Windows users may have to set up an exception for write access # to the hosts file in Windows Security Essentials. hostsFile = '/etc/hosts' startToken = '## start-gsd' endToken = '## end-gsd' siteList = ['reddit.com', 'forums.somethingawful.com', 'somethingawful.com', 'digg.com', 'break.com', 'news.ycombinator.com', 'infoq.com', 'bebo.com', 'twitter.com', 'facebook.com', 'blip.com', 'youtube.com', 'vimeo.com', 'flickr.com', 'friendster.com', 'hi5.com', 'linkedin.com', 'livejournal.com', 'meetup.com', 'myspace.com', 'plurk.com', 'stickam.com', 'stumbleupon.com', 'yelp.com', 'slashdot.com', 'lifehacker.com', 'plus.google.com', 'gizmodo.com'] def rehash(): if sys.platform != 'cygwin': subprocess.check_call(restartNetworkingCommand) def work(): with open( hostsFile, 'a' ) as hFile: print >> hFile, startToken for site in siteList: print >> hFile, "127.0.0.1\t" + site if site.count( '.' ) == 1: print >> hFile, "127.0.0.1\twww." + site print >> hFile, endToken rehash() def play( startIndex, endIndex, lines ): with open(hostsFile, "w") as hFile: hFile.writelines( lines[0:startIndex] ) hFile.writelines( lines[endIndex+1:] ) rehash() if __name__ == "__main__": if sys.platform != 'cygwin' and getpass.getuser() != 'root': exit_error( 'Please run script as root.' ) # Determine if our siteList is already present. startIndex = -1 endIndex = -1 with open(hostsFile, "r") as hFile: lines = hFile.readlines() for i, line in enumerate( lines ): line = line.strip() if line == startToken: startIndex = i elif line == endToken: endIndex = i if startIndex > -1 and endIndex > -1: play( startIndex, endIndex, lines ) else: work()