Rockin' The Geek-Fu, or Not On Your Cloud
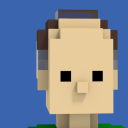
With Foxmarks, you can sync your bookmarks to their servers, or you can sync to your own webdav server. I prefer to host my own stuff, so I sync to my own webdav server. The bookmarks are stored in JavaScript's json file format.
But, that doesn't give me the option to browse to the server and easily see the other set of bookmarks. (If I sync'ed to Foxmarks' servers, then I could see them at my.foxmarks.com if I login with my other username.)
What's a Pythonista to do? Write a script that displays the bookmarks as URLs on a webpage! Sweet! Now I host my own repository for my bookmarks, and they're available online in case I need to see the other set's bookmarks. Here's the script:
#!/usr/bin/python
# foxmarks_reader.py by David Blume
import urllib
import simplejson # http://www.undefined.org/python/
import types
urls = ( ('Home Computer', r'http://user:pwd@url.com/fm/foxmarks.json'),
('Work Computer', r'http://user:pwd@url.com/fmw/foxmarks.json')
)
def do_insert(folders, command):
if 'nid' in command:
args = command['args']
if args.has_key('ntype'):
if args['ntype'] == 'folder':
my_folder = []
folders[command['nid']] = my_folder
if command['nid'] != 'ROOT':
parent = folders[args['pnid']]
parent.append((args['name'], my_folder))
elif args['ntype'] == 'separator':
parent = folders[args['pnid']]
parent.append('---')
else: # 'bookmark'
parent = folders[args['pnid']]
parent.append('<a href="%s">%s</a>' % (args['url'], args['name']))
def print_folders(folders, indent):
for item in folders:
if type(item) == types.ListType or type(item) == types.TupleType:
print '%s%s' % (' '*(indent * 4), item[0].encode('iso-8859-1', 'replace'))
print_folders(item[1], indent + 1)
else:
print '%s%s' % (' '*(indent * 4), item.encode('iso-8859-1', 'replace'))
if __name__=='__main__':
print "Content-type: text/html; charset=ISO-8859-1\n\n"
print '<pre>'
for url in urls:
print '<h2>%s</h2>' % url[0]
sock = urllib.urlopen(url[1])
json = simplejson.load(sock)
sock.close()
folders = {}
for command in json[u'commands']:
do_insert(folders, command)
print_folders(folders['ROOT'], 0)
print '</pre>'
Comments