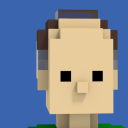
Note to self, next time you mix smart pointers and lambda, remember what to do if things don't resolve automatically. Ex.
#include <iostream>
#include <vector>
#include <boost/shared_ptr.hpp>
#include <boost/lambda/lambda.hpp>
#include <boost/lambda/bind.hpp>
using namespace std;
using namespace boost;
using namespace lambda;
struct B {
explicit B(int a) : a_(a) {}
int get_a() const { return a_; }
private:
int a_;
};
ostream& operator << (ostream &s, const B &b)
{
s << b.get_a();
return s;
}
int main()
{
shared_ptr<B> b1(new B(10));
shared_ptr<B> b2(new B(5));
vector<shared_ptr<B> > v;
v.push_back(b1);
v.push_back(b2);
for_each(v.begin(),v.end(), cout << *_1 << ' ');
cout << endl;
cout << count_if( v.begin(), v.end(), bind(&B::get_a, *_1) > 7);
return(0);
}
Comments